What is Git?
Git is the latest and greatest Version Control System (VCS). The what of a VCS is a piece of software that developers can download onto their computer. They can then interact with it using either the terminal/command line (think stereotypical hacker screen with just green text and typing). More recently, additional software adds a nice UI to the experience. The why of a VCS is to keep track of all of the code changes that are made over time and enable collaboration by multiple developers on the same code sections.
As a comparison, think of writing a recipe for baking cookies, except instead of just one person writing, you have a group of people, each writing one step in the process. First, you create a document with a general outline that includes the preparation, ingredients, and numbered steps. Each person in the group then works on their portion of the recipe. Once everyone finishes writing their step, they give it back to the person who outlined the document, and that person goes through and copies and pastes each section into its appropriate area. A VCS accomplishes this same behavior, but for code.
Let me take the analogy a little further. Working with this many bakers, you can't make just any batch of cookies - the cookies need the right ingredients and process. So for each step, you want to try different options; baking at different temperatures or different lengths of time, adding more sugar, adding less milk, etc. Maybe one variation of a step works better with a variation of another step. It's helpful to keep track of the variations of all the steps, so that you can swap them out and try different combinations.
This collaboration is the core purpose of a VCS. Developers are constantly looking for the best solution to problems, but problems often can be solved with many different orders and combinations. A VCS allows developers to pick out those individual steps and move them around. Or save old versions of a step and reuse them later. Sometimes we change to a step and realize that it completely breaks the whole process, so we have to undo or revert the change altogether.

Why should I care?
Well, if you’re a baker, you probably shouldn’t. On the other hand, if your job involves regular collaboration or even just association with developers, understanding Git can be invaluable. Git has been around since 2005, and since that time, it has taken over the industry. While not every developer will be familiar with Git, it has become so integral to the way we as developers work - that it’s not unreasonable to assume we all should know it.
More and more of the tech industry is becoming dependent on and connected with Git services. More importantly, developers think about programming through the lens of Git. When we talk about what we’re doing, we use Git terminology. We plan how we’re going to work around Git. Even deploying updates to software is frequently done almost entirely through Git. Because of this, it’s beneficial for those interacting with developers to understand the concepts and terminology of Git.
What is a repository?
A repository (repo) is the place where the code is stored. In my baking analogy, the repository is the cookbook or text file where the main recipe is recorded. Each developer has their copy of the code on their computer, but the main copy is stored somewhere online (most of the time) where all developers working on a project can access it. Developers can download a copy of the repository (referred to as “cloning”), make changes on their computer, and upload the changes they made.
Repositories are where names like Github and Bitbucket come into play. These are online services that give developers a place to store their repositories. In my baking example, this would be the cookbook where recipes are stored. Cookbooks sometimes organize recipes into chapters based on the author. Similarly, Github created groups based on teams or users who own specific repositories.
Developers will frequently use the word “repo” for short because five syllables are way too many.

What are branches?
Branches are individual copies of the code. A repository will always have a base branch representing the most up-to-date, approved version of code (usually master or main). In the baking analogy, a branch would be a copy of the recipe. The main recipe that individuals copy and add to or alter. When a developer starts working on a specific task/feature, they pick a copy (branch) and create a new branch off of that. Git then copies everything in the original and puts that into a new branch, allowing the developer to make changes without impacting anyone else’s copies, especially the base branch.
Going back to the baking analogy, say there were fewer people than steps in the recipe. Some people might have to take on multiple steps, but it doesn’t make sense to write all the steps on one copy; you want to see the steps ahead of yours before working on it. So for the first step, you make a copy of the recipe, write out that step, and then hand it back to be copied into the main recipe. For the second step, you do the same thing. Copy the main recipe with all the extra steps from everyone else and add your step. This is the same process developers use. Create a copy (branch), make some changes, copy those changes back into the original, rinse and repeat.
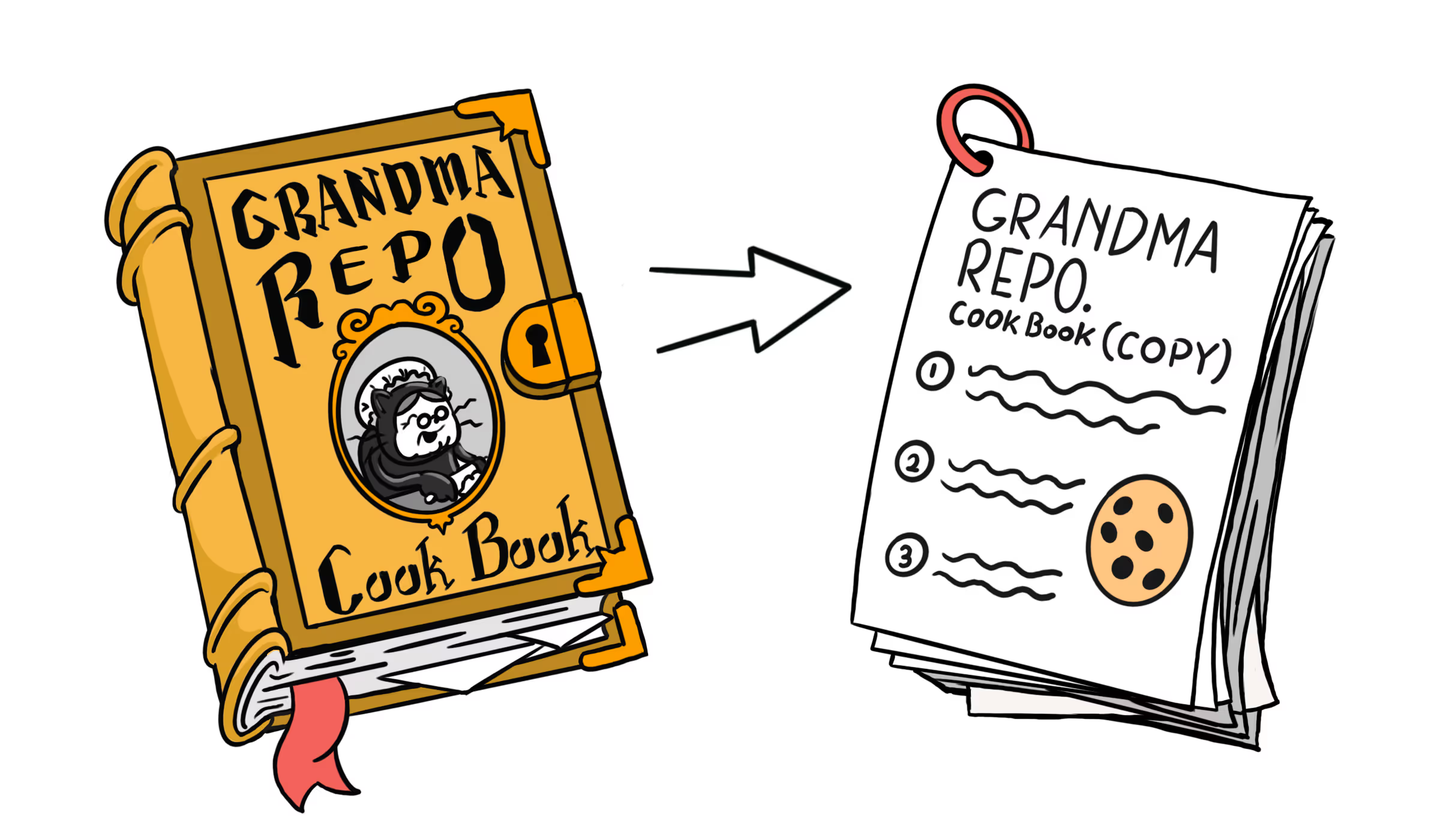
What are feature branches?
Sometimes, there will be longer-standing “feature” branches. These represent a change that requires multiple pieces to get to the intended feature. Compared with the baking analogy - this would be like if you decide to change the recipe from cookies to brownies but realize the steps are very similar. You could create a “brownies” feature branch and create branches off that to make changes to each step.
Initially, you want to keep those changes separate from the main cookie recipe, or you’ll end up with a Frankenstein cookie-brownie recipe. Eventually, you’ll want to replace the cookie recipe with the brownie recipe in its entirety. While individual steps in the recipe may not change, the whole recipe needs to uniformly illustrate how to make brownies, not cookies.
This recipe branch is the same idea behind feature branches. Something fundamental needs to change about the code, and it can’t be done as iteratively, step-by-step, as the normal process. A feature branch is created to keep all those changes separated until everything works together and can move back to the base branch.
What is merging?
Merging is exactly what it sounds like. After a developer has created a new branch, made changes that (seem) to work, they need a way to get those changes back to the original branch. To do this, we would “merge” our branch into the original. Git then goes through and replicates changes made to the original. In the baking analogy, this would be the process of copying over each person’s step into the main recipe.
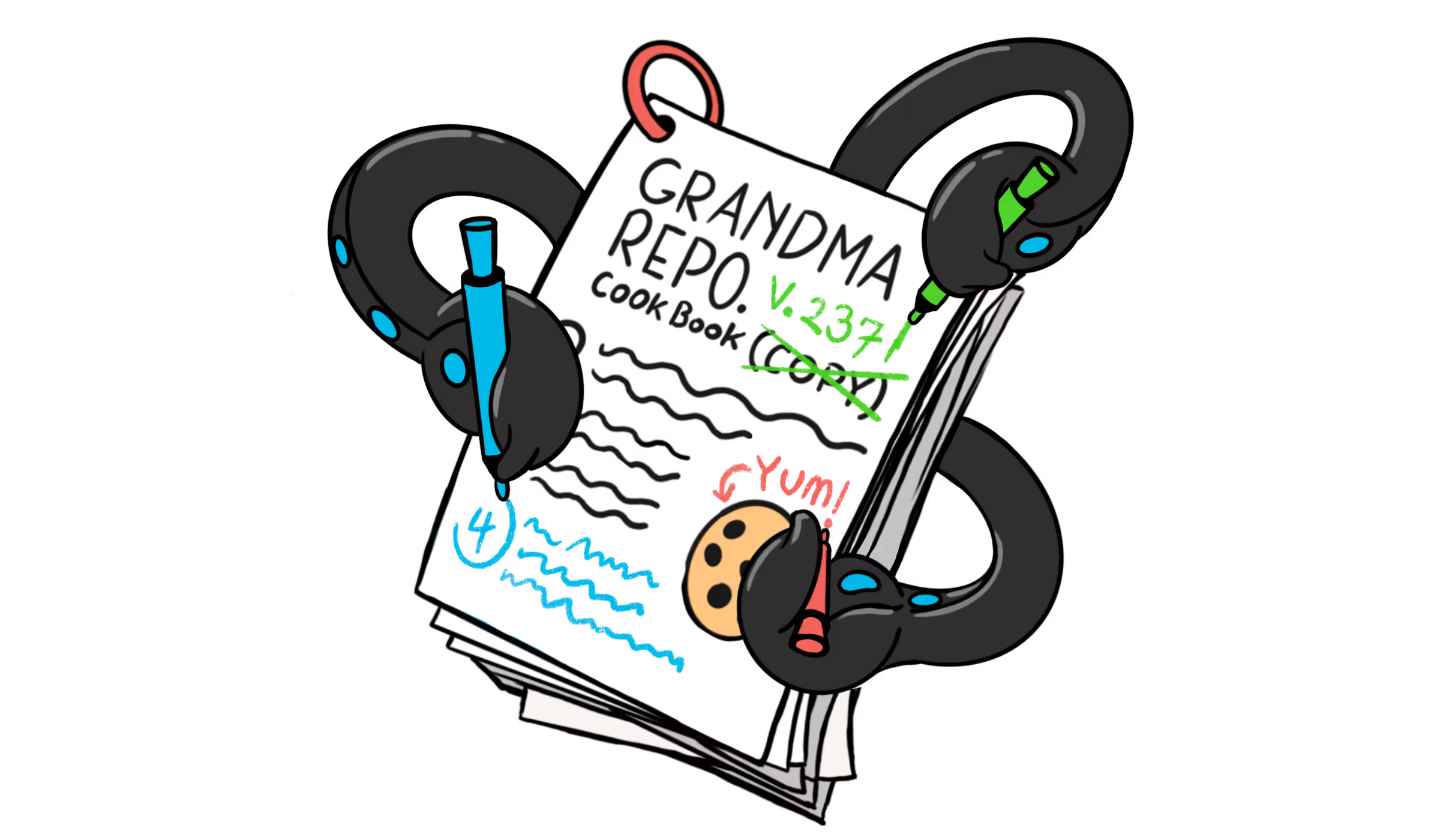
What is a commit?
A commit is how a developer saves a set of changes to Git. Once a developer has reached a stopping point, the developer will commit the changes. Git then saves these changes to the branch they’re currently working on. After that, the developer can switch to a different copy of the code branch, and when they come back, their changes will still be there.
Having commits is incredibly powerful because it allows developers to see every incremental change made to the code - enabling us to identify where an issue originated. Changes made can be reversed or undone and even applied to other branches.
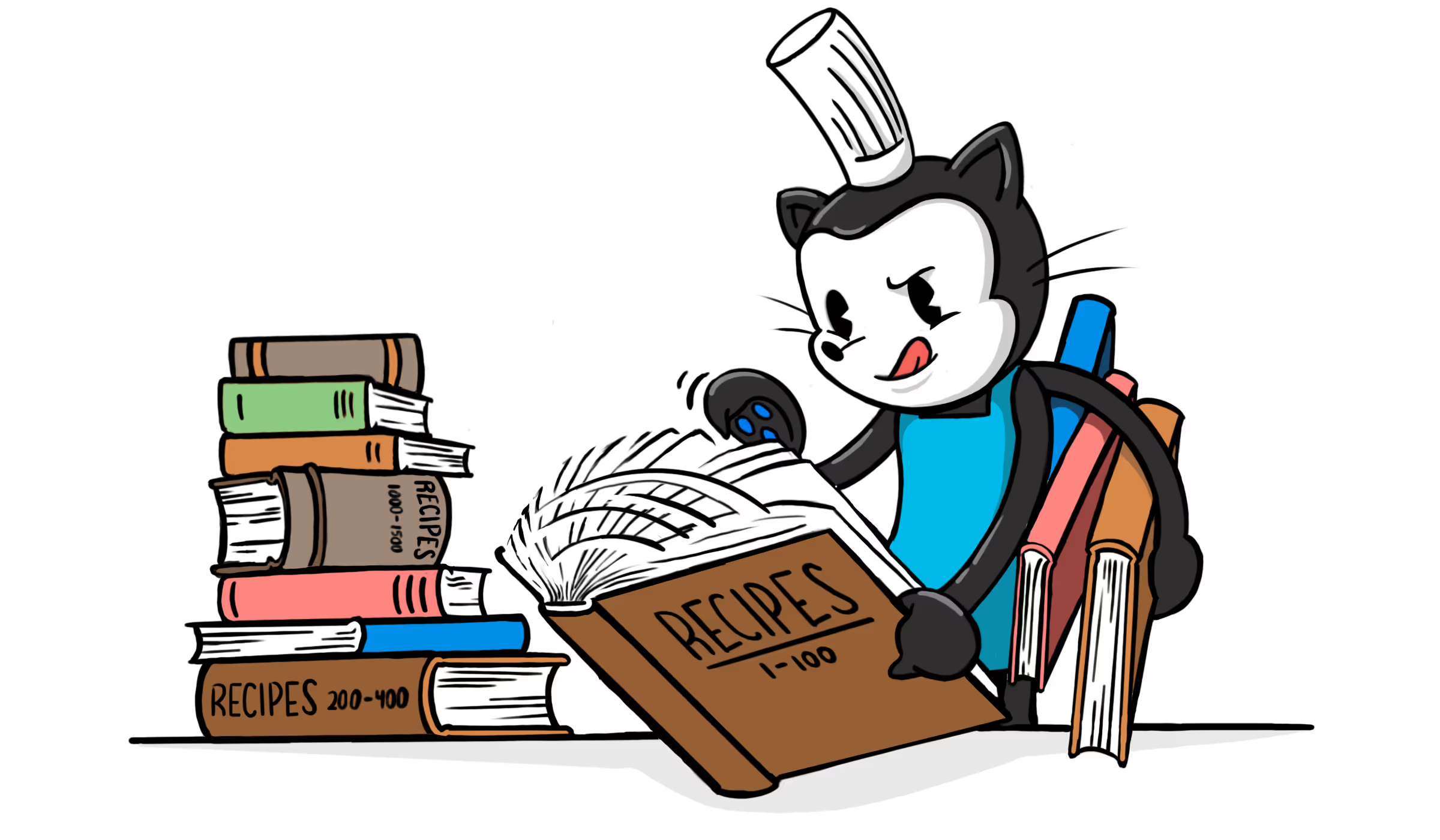
What does it mean to push?
As I mentioned above, code is stored in a repository, but developers can copy their versions of code (branches) and make changes independently. Pushing is when each developer adds their version of code to the repository. Compared with the baking analogy, this would be the process of handing in your copy of the recipe.
When a new version of code is pushed, it creates a new branch in the repository. If there is an existing branch with changes, it will overwrite the branch with the updated changes. Git is adding all of the new commits (savepoints) to the repository branch. This way, a developer can easily undo a change made in the repository by removing a commit from their branch and pushing their changes to the repository.

Can you pull?
Of course, pulling is the term used to describe downloading a repository’s version of the code. Equivalent to pushing, this can either overwrite an existing branch or create a new one. Compared with the baking analogy, this would be the process of copying changes that have happened to the main recipe onto your copy.
Most likely, your step impacts others and visa versa. For example, if you’re writing the step for preparing the cookies after they’ve been baked (like frosting them), it’s helpful to know if the previous step has suggested waiting to let the cookies cool before continuing. We could reasonably list this information in both steps. Previous steps influence what you need to write in yours. Coding is very similar, and in most cases, each step impacts most if not all of the other steps. Pulling allows us to capture all of the changes pushed through.
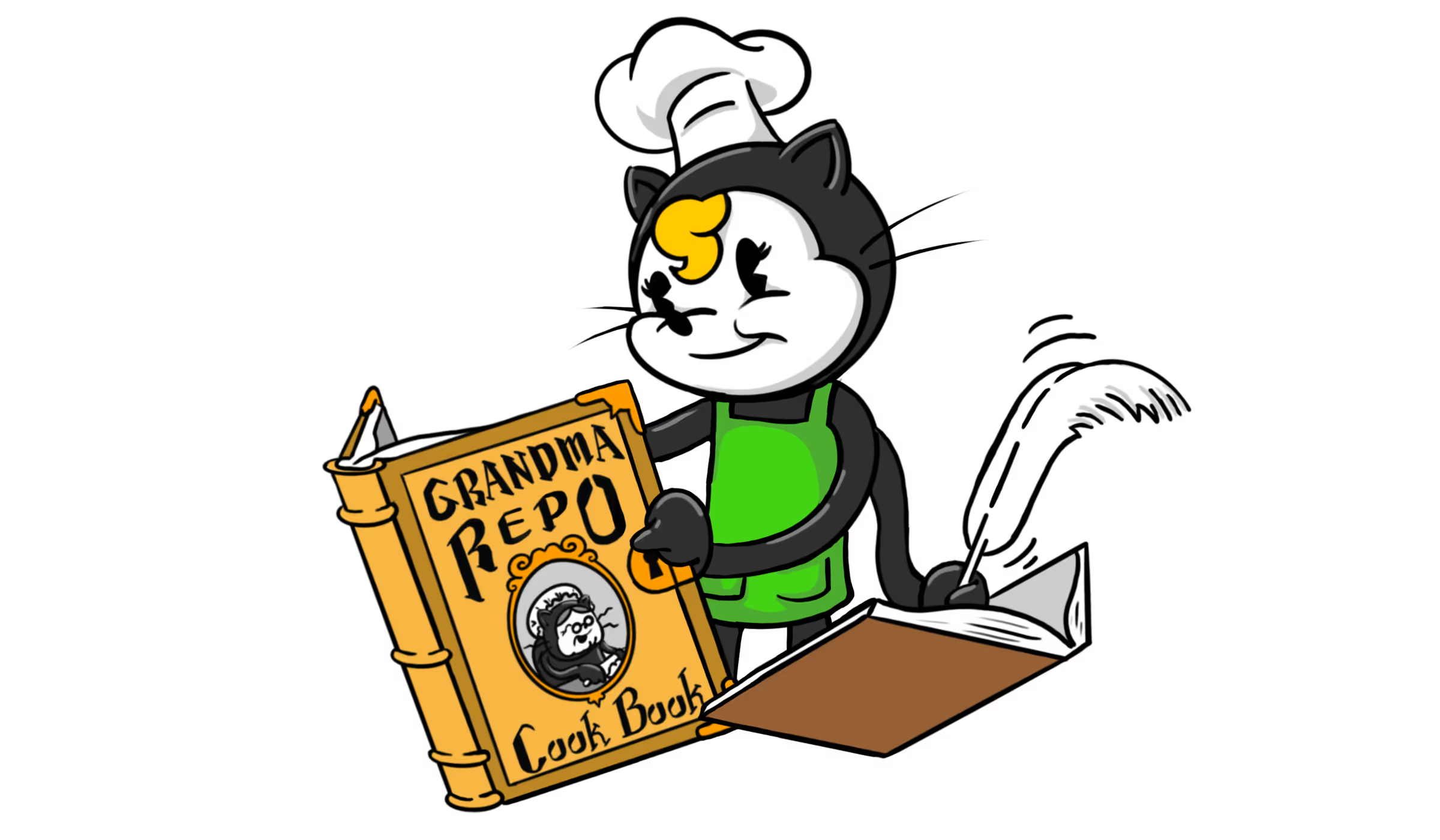
What’s the difference between Git and Github?
In addition to describing Github in the repository section, I wanted to call specific attention to this because I know it can be a frequent point of confusion. Github is one of many repository services; it’s a place where the code is stored. Git is the software used by developers to do everything else described here. Git is used to interacting with a Github repository by pushing and pulling.
What else?
Git is a powerful and complex piece of software. There are a lot of features not described in this article (and honestly quite a few that I am not familiar with), but understanding these aspects of it should be enough to help you in conversations with developers. If you’re interested in learning more about Git, there are a lot of resources online, but from my research, most are targeted to developers, so they might be difficult to follow. Maybe you could ask your friendly neighborhood developer. We’re usually nerds that love talking about nerdy things.